Helpful Support Tools from ActiveSupport
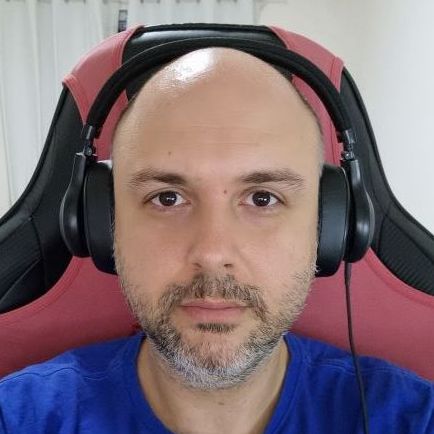
If you have already dug down inside the Rails code, you certainly saw something about ActiveSupport. Today I’ll present to you my two favourite tools that this library can offer to us.
CurrentAttributes
ActiveSupport::CurrentAttributes
can store values and allow you to access them from any place in your code. It’s thread-safe, and resets per requests. Recently I thought of using it on a use case where I needed to define what will be the chosen DB shard that will receive the data by the user id. Let’s build an idea of how it could work:
```ruby
# app/models/current_shard.rb
class CurrentShard < ActiveSupport::CurrentAttributes
attribute :user, :shard
end
# app/controllers/concerns/set_current_shard.rb
module SetCurrentShard
extend ActiveSupport::Concern
included do
after_action :authenticate
end
private
def choose_shard
if current_user
CurrentShard.user = current_user
CurrentShard.shard = ShardIdentifier.call(current_user.id)
end
end
end
class ApplicationController < ActionController::Base
include SetCurrentShard
before_action :set_shard
private
def set_shard
ActiveRecord::Base.connected_to(role: :writing, shard: CurrentShard.shard) do
yield
end
end
end
Using this idea, the application could rely on the shard that was defined after the authentication flow. All the requests will always have a shard definition before the execution of any DB operations. Pretty fun!
Configurable
This tool allows us to store some configuration for an object. It adds a config method to which we can set the configs.
```ruby
class Example
include ActiveSupport::Configurable
end
Example.config do |config|
config.any_option = 'nice info'
end
Example.config.any_option
=> 'nice info'
It’s super simple and flexible. You can use it to customize the behaviour of your gem, handle global configurations on your application, or define configuration for any Ruby object. It’ll be a good choice for any circumstance where you need to configure classes dynamically.
Other nice tools
The ActiveSupport
has more good tools for us if we continue digging. Like the Callbacks
, MessageVerifier
, or the MessageEncryptor
. If you want to see more about it, let me know. :)
See you on my next blog here friends!